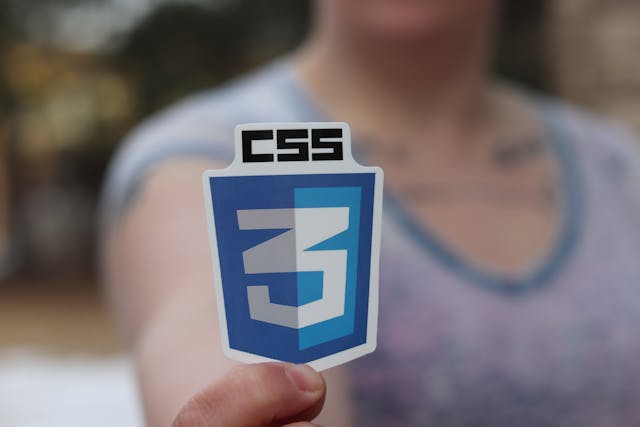
CSS
Basics
-
CSS stands for Cascading Style Sheets
-
CSS describes the visual style and presentation of the content written in HTML
-
CSS consists of countless properties that developers use to format the content: properties about font, text, spacing, layout, etc.
Example (A CSS rule)
h1 { color: blue; text-align: center; font-size: 20px; }
- h1 -> Selector
- {...} -> Declaration block
- font-size: 20px; -> Declaration/Style
- font-size -> Property
- 20px -> Value
3 ways of writing CSS
- inline CSS (should never be used)
<h1 style="color:blue">The Code Magazine</h1>
- Internal CSS (inside head tag)
<style> h1 { color: red; } </style>
- External CSS (in a style.css file and link it inside the head tag)
<link href="../public/css/style.css" rel="stylesheet" />
CSS theories
#1 Conflicts between selectors
<p id="author-text" class="author"> Posted by Laura Jones on Monday, June 13 2023 </p>
.author { font-style: italic; font-size: 18px; } #author-text { font-size: 20px; } p, li { font-family: sans-serif; color: #444444; font-size: 22px; }
- There are multiple selectors selecting the same element, which one of them applies?
- All of them. All rules and properties applied.
- But there are conflicting font-size declarations. Is it 18px, or 20px or 22px?
highest priority --------> lowest priorities (5) ↓ Declarations marked !important (4) ↓ Inline style (style attribute in HTML) (3) ↓ ID (#) selector (2) ↓ Class (.) or pseudo-class ( : ) selector (1) ↓ Element selector (p, div, li, etc.) (0) ↓ Universal selector (*)
#2 Inheritance
How inheritance works?
<body> <nav> This is a navigation </nav> <h1>My website</h1> <p> The text in this paragraph is completely irrelevant </p> </body>
body { color: #444444; font-size: 16px; font-family: sans-serif; border-top: 10px solid #1098ad; } h1 { color: #1098ad; font-size: 32px; text-transform: uppercase; }
- The border property DOES not get inherited.
Not all properties get inherited. It's mostly ones related to text: font-family, font-weight, font-style, color, etc.
- h1 { styles } are OVERRIDING inherited styles.
#3 CSS Box Model
Layers of the CSS Box Model
- Content: Text, images, etc.
- Border: A line around the element, still inside the element
- Padding: Invisible space around the content, inside the element
- Margin: Space outside the element, between elements
- Fill area: Area that gets filled with background color or background image
Element height and width calculation
- Final element width = left border + left padding + width + right padding + right border
- Final element height = top border + top padding + height + bottom padding + bottom border
We can specify all these values using CSS properties This is the default behavior, but we can change it
Collapsing margins Margin collapsing
The top and bottom margins of blocks are sometimes combined (collapsed) into a single margin whose size is the largest of the individual margins (or just one of them, if they are equal), a behavior known as margin collapsing. Note that the margins of floating and absolutely positioned elements never collapse.
#4 Types of Boxes
Block level elements
- Elements are formatted visually as blocks
- Elements occupy 100% of parent element's width, no matter the content
- Elements are stacked vertically by default, one after another
- The box model applies as showed earlier
Default elements: body, main, header, footer, section, nav, aside, div, h1-h6, p, ul, ol, li, etc. With CSS: display: block
Inline level boxes
- Occupies only the space necessary for its content
- Causes no line-breaks after or before the element
- Box model applies in a different way: heights and widths do not apply
- Paddings and margins are applied only horizontally (left and right)
Default elements: a, img, strong, em, button, etc. With CSS: display: inline
Inline-block level boxes
- Looks like inline from the outside, behaves like block-level on the inside
- Occupies only content's space (same as inline-level boxes)
- Causes no line-breaks (same as inline-level boxes)
- Box model applies as showed (same as block-level boxes)
- With CSS: display: inline-block
#5 Absolute positioning
Normal flow | Absolute positioning |
---|---|
Default positioning | right-aligned |
Element is "in flow" | Element is removed from the normal flow: "out of flow" |
Elements are simply laid out according to their order in the HTML code | No impact on surrounding elements, might overlap them |
We use top, bottom, left or right to offset the element from its relatively positioned containers | |
position: relative | position: absolute |